
Discover the step-by-step guide to creating an AI-powered profile photo verification system with EyePop.ai and JavaScript. Perfect for beginners, this tutorial will help you implement a robust verification process, improving the quality and relevance of user-uploaded images on your platform.
Revolutionizing Profile Photo Verification in 10 Minutes with EyePop.ai and JavaScript

In the digital era, ensuring the quality and relevance of user-uploaded photos has become a challenge for many platforms. Whether it's for a social media profile, a community forum, or a user verification process, maintaining a high standard for uploaded images is essential. That's where the magic of AI, particularly EyePop.ai, combined with the versatility of JavaScript, comes into play.
Today, I'm excited to share how you can build a sophisticated profile photo verification system in just 10 minutes. Yes, you heard it right – in less time than it takes to make your morning coffee! ☕✨
EyePop.ai makes computer vision effortless. Sign up free.
Introducing EyePop.ai: Democratizing Computer Vision
EyePop.ai is at the forefront of this revolution, making advanced computer vision available to everyone. Whether you're a developer, a business owner, or a coach, EyePop.ai provides the tools you need to harness the power of AI-driven insights, without requiring a PhD in computer science.
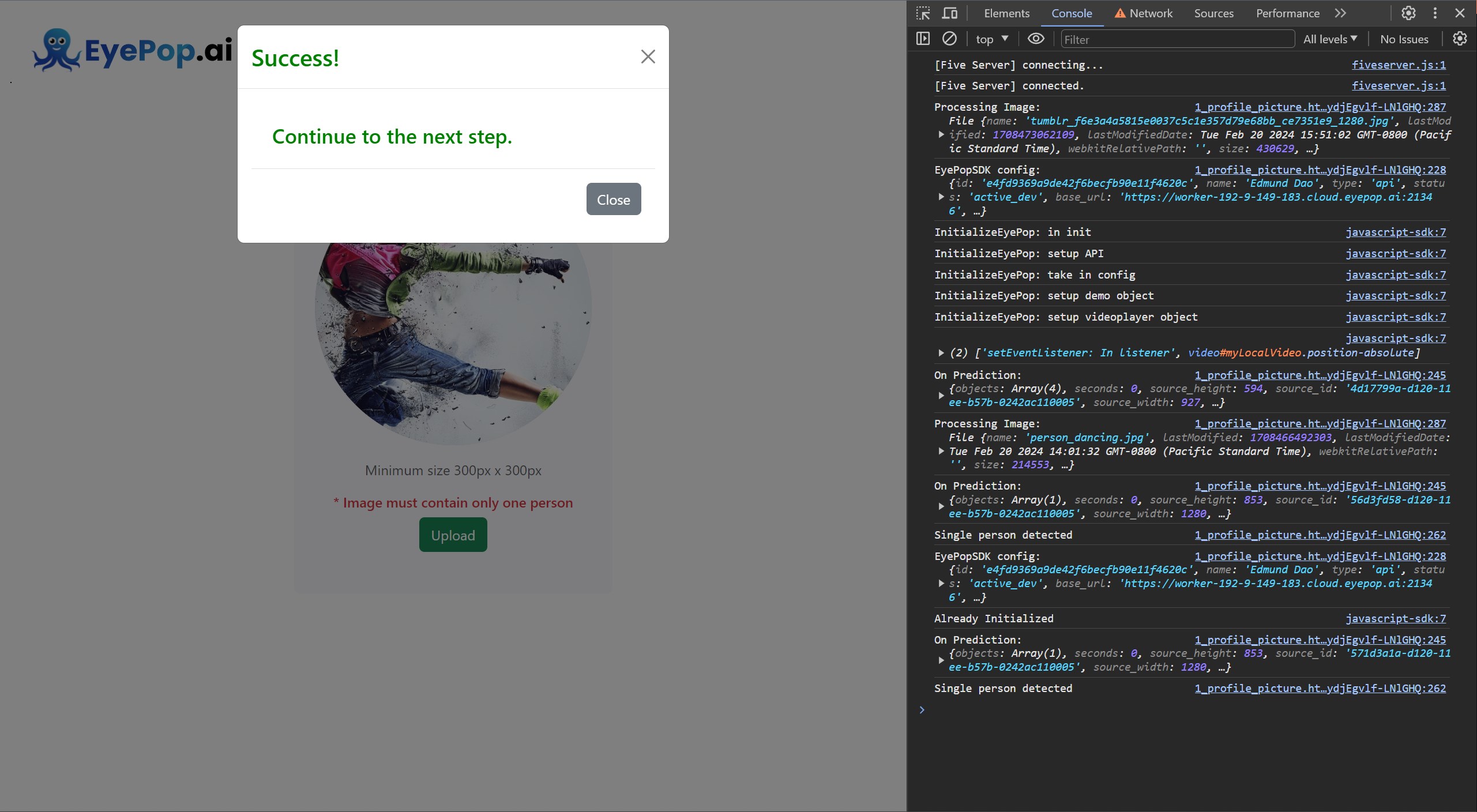
Step-by-Step Guide to Profile Photo Verification
Now, let's dive into the heart of our discussion: building a profile photo verification system. The process involves a few simple steps below and requires web development knowledge.
We'll skip the simple HTML page design and show how simple using the EyePop SDK is.
Signup for Free!
- Follow this quick guide to setup an API Pop in the developer docs.
- Setup your webpage and user image upload workflow, we'll skip those details here but feel free to clone the Demo repo and use the following site provided there!

- Hook up your Pop in just a few lines of Javascript, enter your Pop UUID and EyePop API Key here. (Note: Some boilerplate HTML is required also, such as a canvas with an id of "mobilecanvas" and a video with id of "localVideo", and file upload element with an id of "file_upload". Our SDK is constantly being upgraded, so expect changes and improvements!)
- Next, add the following code to detect when a single person is in an image!
That's it! Your profile verification system is ready to go! The possibilities are endless, check out more demos here: https://github.com/eyepop-ai/Demos